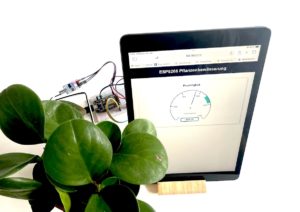
Pflanzenbewässerung mit einem ESP8266 Webserver
In einem früheren Projekt habe ich eine automatische Pflanzenbewässerung mit einem Arduino UNO vorgestellt. In diesem Projekt hier gehst du einen Schritt weiter: Du baust
In einem früheren Projekt habe ich eine automatische Pflanzenbewässerung mit einem Arduino UNO vorgestellt. In diesem Projekt hier gehst du einen Schritt weiter: Du baust
Für einige Projekte ist es praktisch, Dateien auf einem ESP8266 zu speichern – z.B. weil du Sensordaten sichern möchtest, oder auch die HTML-, CSS- und
Möchtest du mit deinem ESP8266 oder ESP32 Nachrichten verschicken, um zum Beispiel eine Warnung von einem Bewegungsmelder oder Temperatursensor zu erhalten? In diesem Tutorial erfährst
Baue eine ESP8266 Wetterstation, die dir die aktuelle Temperatur, Luftfeuchtigkeit und den Luftdruck anzeigt sowie deine Daten speichert und visualisiert. Die aktuellen Messdaten erscheinen auf
Der ESP8266 ist ein vielseitiger und preiswerter Mikrocontroller mit integrierter WiFi-Funktionalität. Eine seiner bemerkenswertesten Funktionen ist jedoch der Deep-Sleep-Modus, der es ermöglicht, den Stromverbrauch drastisch
Wenn du an einen Raketenstart denkst, kommt dir bestimmt vieles in den Sinn. Sicherlich auch der obligatorische Countdown. Wie wäre es, wenn du daheim die
Gehst du ab und an vergeblich zum Briefkasten? Das ist jetzt vorbei! Dieses Projekt sendet dir eine Telegram-Nachricht aufs Smartphone, sobald der Briefträger die Post
In diesem Projekt lädst du dir mit einem ESP32 aktuellen Schlagzeilen aus dem Internet und findest mit einer Sentimentanalyse heraus, ob sie positiv oder negativ
Du hast eine LEGO Mondlandefähre (Apollo 11 Lunar Lander), aber dir fehlt noch die richtige Beleuchtung? In diesem Projekt verbaust du unter der Landefähre einen
In diesem Projekt lernst du, wie du von deinem Smartphone aus eine Lichtquelle ein- und ausschalten kannst. Außerdem kannst du von dort aus abfragen, ob
Mit der ESP32-CAM kannst du einfach und günstig deine Kameraprojekte umsetzen, wie z.B. unsere Fotofalle. Aber es müssen ja nicht immer nur Fotos sein –
In diesem Projekt verwendest du Telegram, um bei deinem ESP8266 nachzufragen, wie hoch die aktuelle Temperatur ist. Sobald dein ESP8266 deine Anfrage erhalten hat, fragt
In diesem Projekt überwachst du die Temperatur mit einem Sensor und deinem ESP8266. Sobald der Temperatursensor einen von dir festgelegten Wert ermittelt, sendet dein Microcontroller
Hinweis: Leider ist dieses Projekt bis auf Weiteres nicht mehr umsetzbar, da die benötigte API zur Ermittlung der nächsten Überflüge nicht mehr online ist. Du
ESP32- und ESP8266-Boards sind zusammen mit Telegram eine tolle Kombination. Du kannst Daten blitzschnell auf dein Smartphone senden und von dort aus deinen Microcontroller steuern.
In diesem Projekt baust du dir einen Bewegungsmelder, der dir eine Nachricht schickt, wenn sich vor ihm jemand bewegt. Im Prinzip handelt es sich hierbei
In diesem Projekt baust du dir mit einem ESP8266 einen Dash Button, der dir auf Knopfdruck eine Nachricht an deinen Telegram Account sendet. Du kannst
Wenn du mit deinem ESP8266 oder ESP32* oder auch mit dem Arduino im Internet bist, dann sicher nicht ohne Grund. Vielleicht möchtest du Daten von
Bluetooth ist allgegenwärtig und auch dein ESP32 kann damit umgehen. Lerne in diesem Tutorial, wie du Bluetooth Classic verwendest und Daten zwischen einem Smartphone und
In diesem Projekt baust du dir eine Alarmanlage, die dir eine E-Mail schickt, sobald sich in ihrer Nähe etwas bewegt, das eigentlich nur von dir
Hier erfährst du, was MicroPython ist, welche Vorteile dir diese Programmiersprache bietet und wie du sie auf deinem ESP8266* oder ESP32* installierst. Was ist MicroPython?
Der Microcontroller ESP8266 eignet sich nicht nur perfekt, um Daten aus dem Internet abzurufen und zu verarbeiten. Mit ein paar Zeilen Code baust du dir
Wenn du mit einem ESP8266* arbeitest und ohnehin damit im Internet bist, gibt es eine einfach Möglichkeit, die aktuelle Uhrzeit herauszufinden: Mit der Bibliothek NTPClient.h
Stoße die Tür zum Internet of Things weit auf! Wenn du für dein Projekt eine Verbindung zum Internet benötigst, leisten dir die beiden Controller ESP8266*
Du postest Fotos und Videos auf Instagram? Aber Notifications zu neuen Likes auf deinem Smartphone sind dir zu langweilig? Dann probiere etwas Neues aus! Mit
Hast du schon einmal deine Wohnung verlassen und bist dann auf der Straße im Regen gestanden? Dafür, dass das nicht noch einmal passiert, sorgst du
Du möchtest die neuesten Schlagzeilen direkt in deinem Seriellen Monitor lesen? Dann wird es Zeit für deinen eigenen Newsticker! In diesem Projekt verbindest du deinen
Baue spannende Projekte mit deinem ESP8266 und stoße die Welt zum Internet of Things auf! Diese Microcontroller sind günstig, passen (in der Amica-Version, mehr dazu weiter unten) auf jedes Breadboard und können oft auch einen Arduino ersetzen – auch wenn du eigentlich keine Internetverbindung benötigst.
Das beste Argument für einen ESP8266 ist sein WLAN-Modul, denn mit ihm bist du nicht mehr auf dein Arbeitszimmer oder deine Werkstatt beschränkt: Spielend leicht verbindest du dein Projekt mit dem Internet und erschließt dir ganz neue Möglichkeiten!
Dazu gehören zum Beispiel:
Die Vorteile liegen auf der Hand: Der ESP8266 ist preiswert und sogar ganz einfach über die Arduino IDE zu programmieren. Wie das geht, lernst du in diesem Tutorial. Viele Projektideen kannst du ohne allzu viel Aufwand umsetzen, da es bereits für viele Probleme Bibliotheken gibt, die du einfach integrieren kannst. Wenn du noch mehr Power und vielleicht sogar Bluetooth brauchst, dann ist vermutlich der große Bruder ESP32 der nächste Kandidat auf deiner Liste.
Beim ESP8266 stehen dir ähnlich viele Pins zur Verfügung wie beim gewohnten Arduino. Du hast die Möglichkeit, eine ganze Reihe Sensoren, Display oder andere Bauteile per I²C ( Inter-Integrated Circuit) anzuschließen. Auch die Kommunikation via SPI (Serial Peripheral Interface) ist kein Problem. Hinzu kommen eine ganze Reihe digitale Ein- und Ausgänge sowie ein Pin, um analoge Signale zu lesen.
Deiner Kreativität steht somit nichts im Wege: Wie wäre es mit einer Wettervorhersage? Oder mit einem Counter für deine Follower auf Instagram? Oder lass dir eine E-Mail schicken, wenn die Luft in deinem Arbeitszimmer zu dick ist. :)
Wenn du mit den Pins deines Arduinos vertraut bist, dann fällt dir das Experimentieren mit einem ESP8266 Projekt nicht schwer. Der Controller bietet dir zahlreiche Pins für die digitale Kommunikation. Ebenso kannst du analoge Daten auslesen, denn ein Analog-Digital-Wandler ist auch an Bord!
SPI und I²C sind auch kein Problem. Somit kannst du auch mehrere Komponenten anschließen, ohne haufenweise Pins zu belegen und Kabel anzuschließen.
Alles in allem hast du mit dem ESP8266 einen Microcontroller, der eigentlich mehr kann als ein “normaler” Arduino und den du deshalb vielleicht schon bald bevorzugen wirst.
Wenn du im Internet auf der Suche nach einem ESP8266 bist, begegnest du in der Regel zwei Versionen: dem NodeMCU Amica (v2) und der NodeMCU LoLin (v3). Obwohl eine v3 eigentlich für eine Verbesserung spricht, ist das nicht unbedingt der Fall. Im Gegenteil, die LoLin-Version hat einen gravierenden Nachteil: Sie passt zwar auf dein Standard-Breadboard, allerdings hast du dann keinen Platz mehr, um Kabel neben die Pins des ESP8266 zu setzen.
Dieser Umstand macht das Bauen an Prototypen unnötig komplizierter. Im Gegenzug sind die Vorteile der v3 gegenüber der v2 – also der Amica-Version – marginal und für die meisten Maker nicht so wichtig.
Wir empfehlen dir deshalb für deine ESP8266 Projekte den Kauf eines “Amica”.
Diese beiden Namen tauchen manchmal zusammen auf, manchmal aber auch nur der letzte. Auch wir bei pollux labs verwenden in der Regel immer nur “ESP8266”. Gemeint ist eigentlich immer ein und derselbe Microcontroller.
Aber um genau zu sein: NodeMCU ist das Betriebssystem dieses Boards und wurde im Jahr 2014 entwickelt. Der ESP8266 hingegen ist der Microcontroller auf dem dieses Betriebssystem läuft.
Neue Projekte und Tutorials in deinem Postfach.